Flutter : Integrate to Firebase Faster with FlutterFire CLI
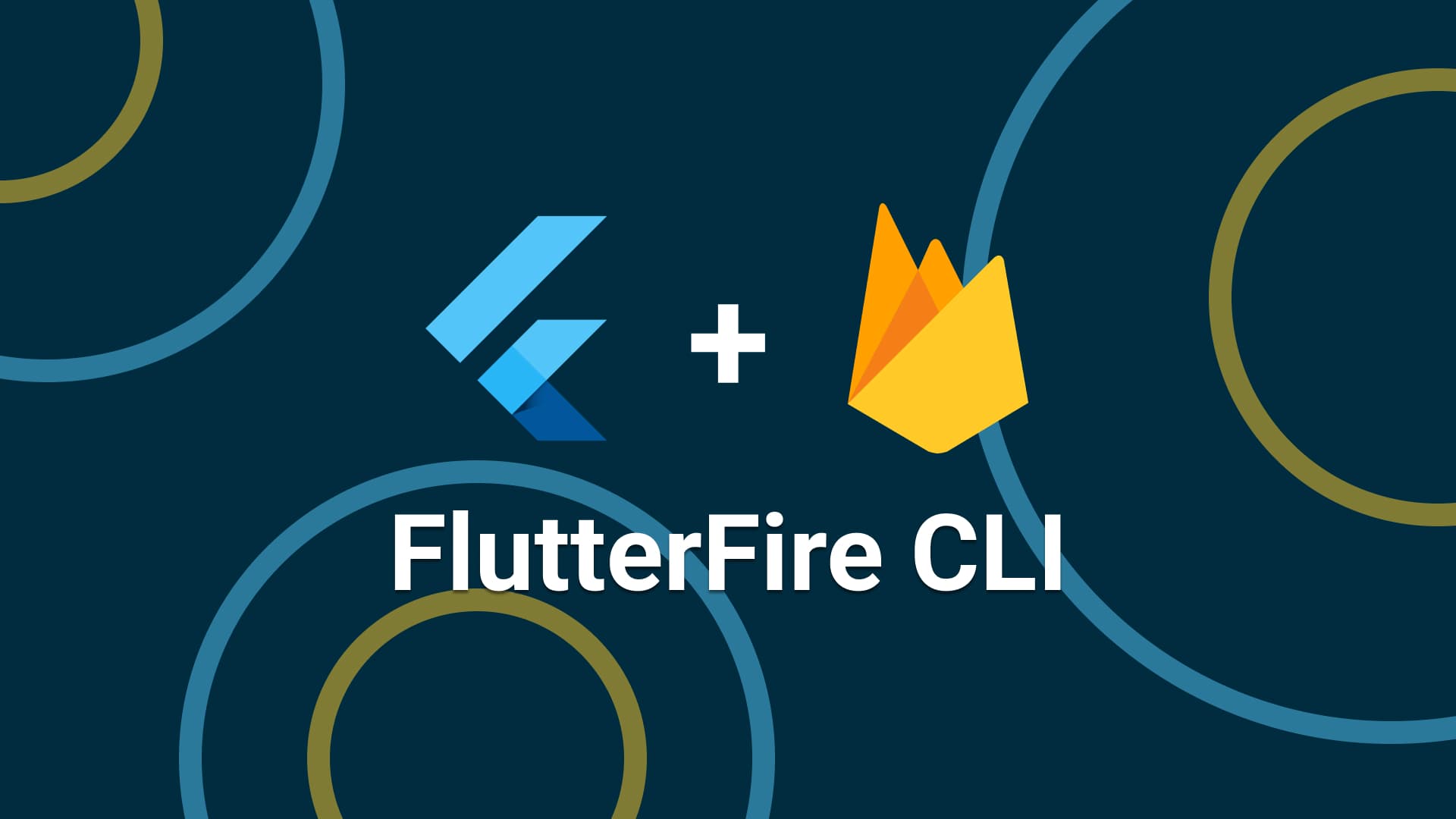
Firebase is one of google's product which has many feature to make apps development process more easier. It looks like software-as-service with their ability in build, monitoring, messaging, etc also backend-as-service which we can use their realtime nosql database, authentication, storage, etc. We can use all this service to many platform like android, ios, web, and desktop. Of course to use all this feature, we need to integrate it first and it takes a lot of time if we integrate it manually. So, we will use FlutterFire CLI to make our integration between flutter and firebase faster. Why we need FlutterFire ??? Because flutter is multiplatform project, we must create project one by one each platform in firebase if we integrate it manually. this CLI can do this boring task just in some command. Lets learn how to use it.
1. Create Flutter Project
Create your Flutter Project like you always do. You can create it by Android Studio and command in terminal like below.
flutter create flutter_project_name
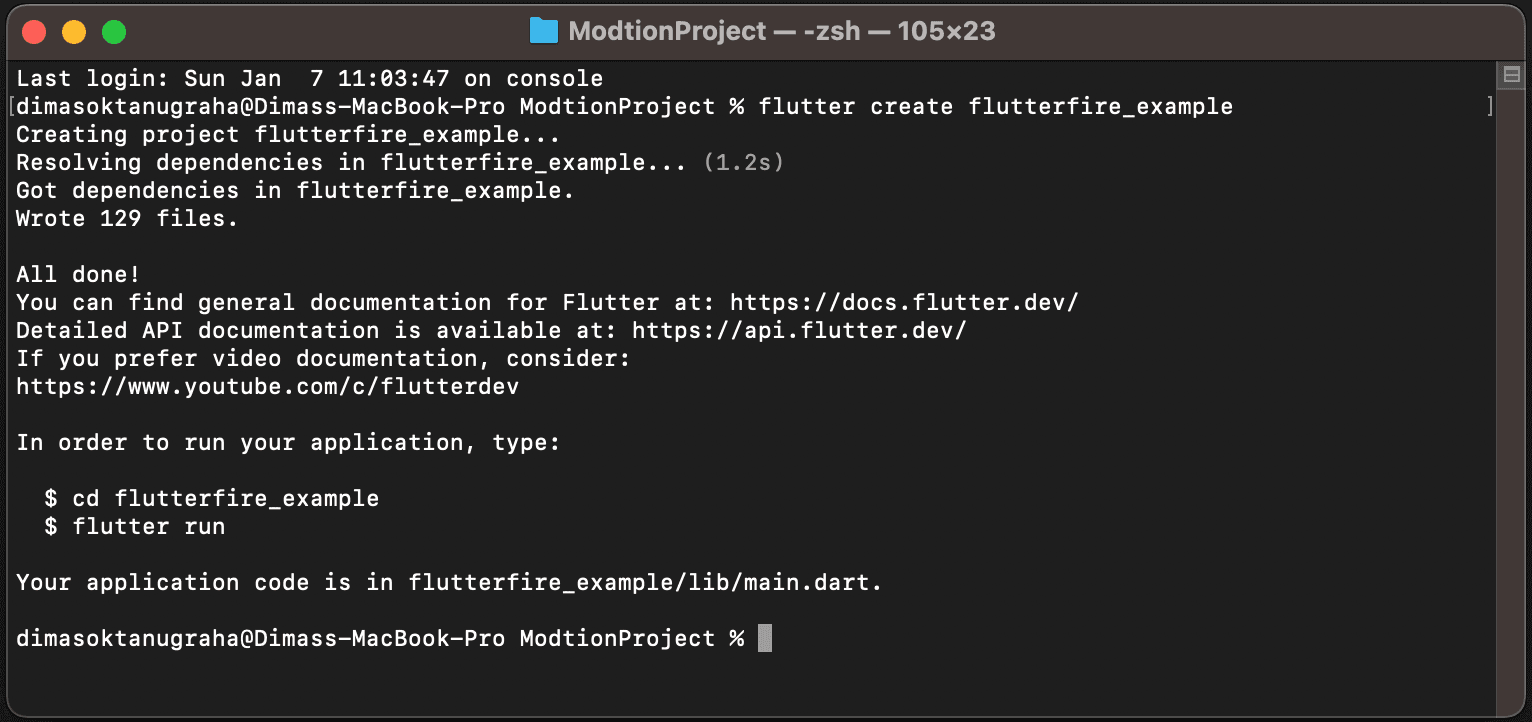
2. Create Firebase Project
Create your Firebase Project. Actually you can new project from CLI too, but for now we can create it manually in firebase console with your account. Follow this step one by one:
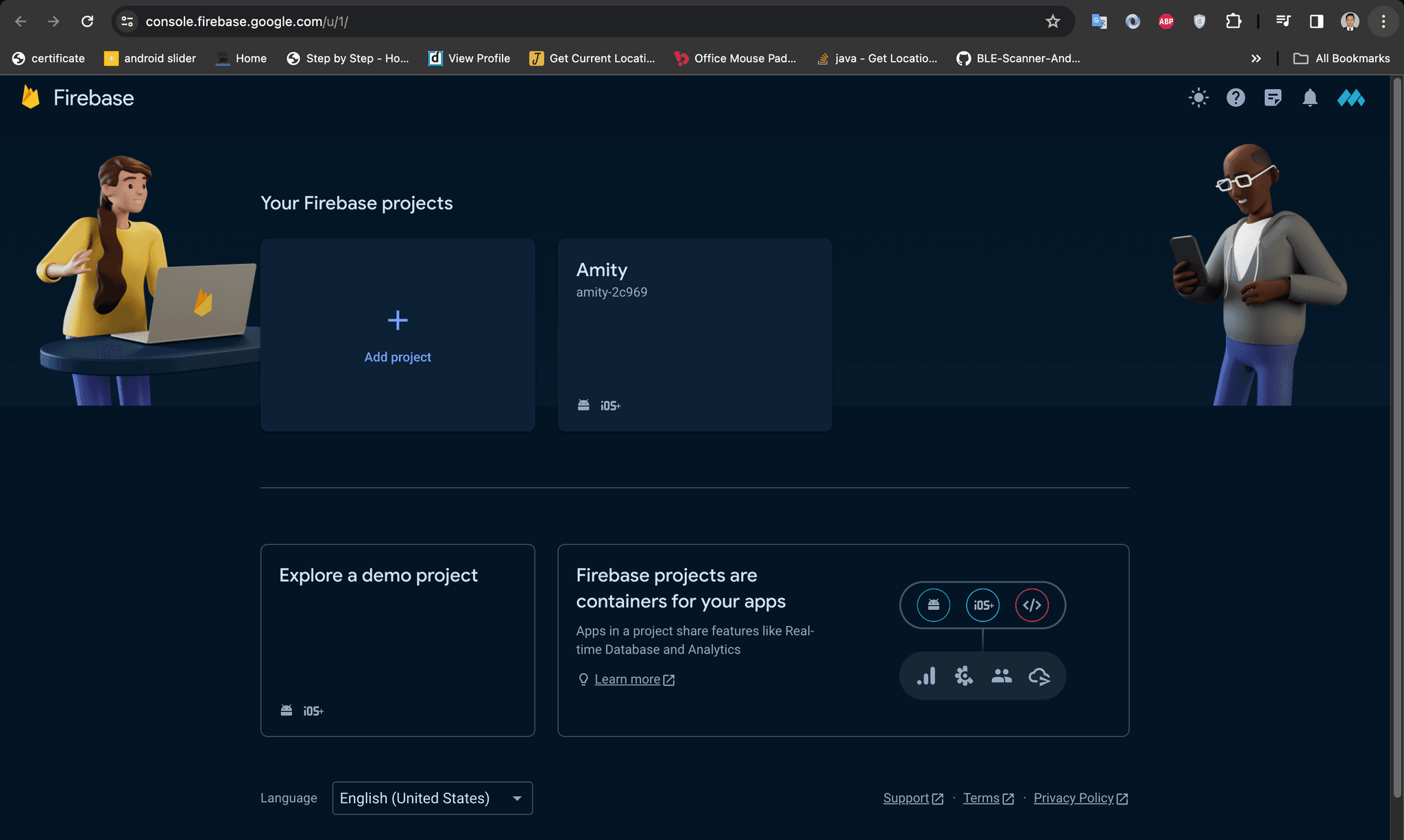
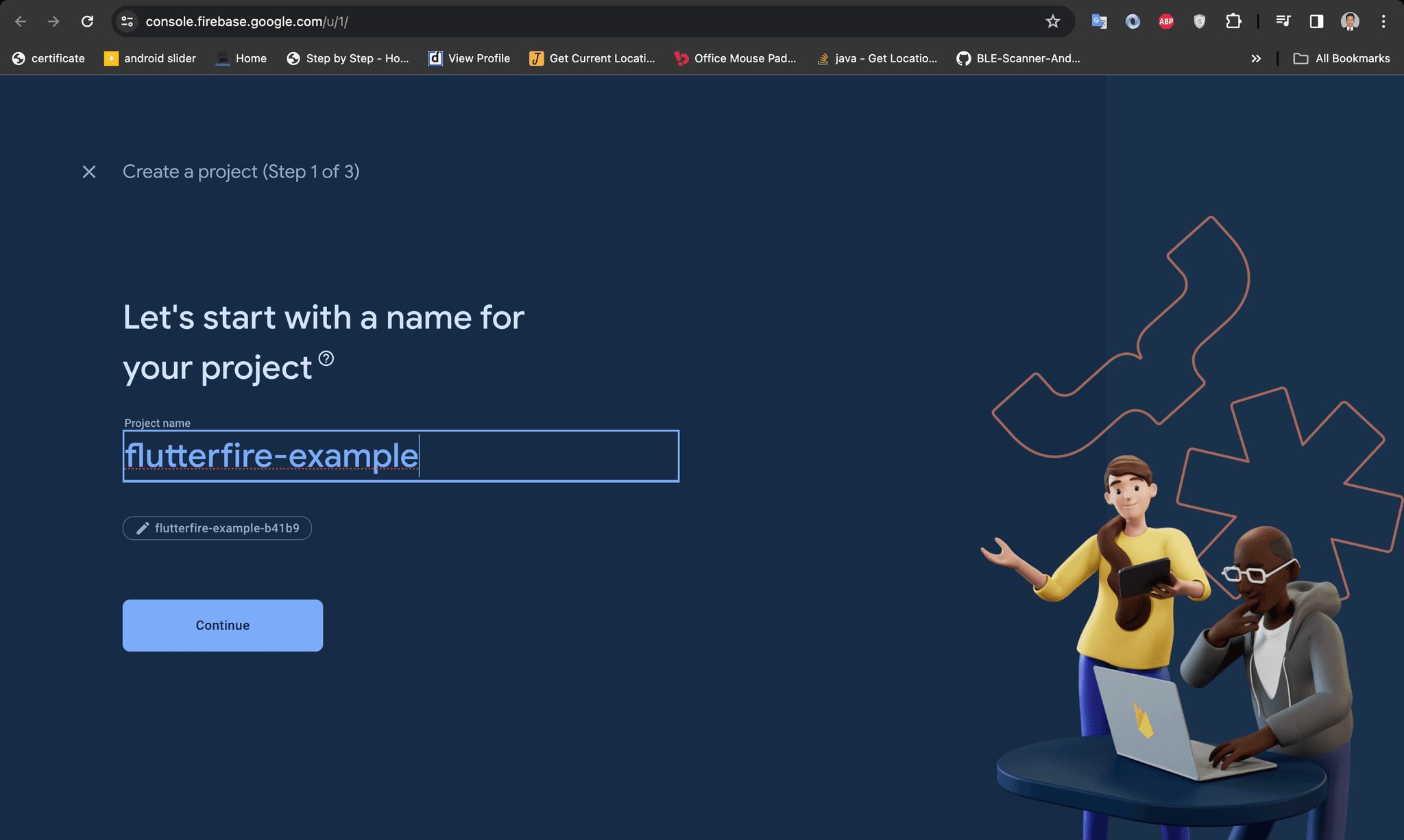
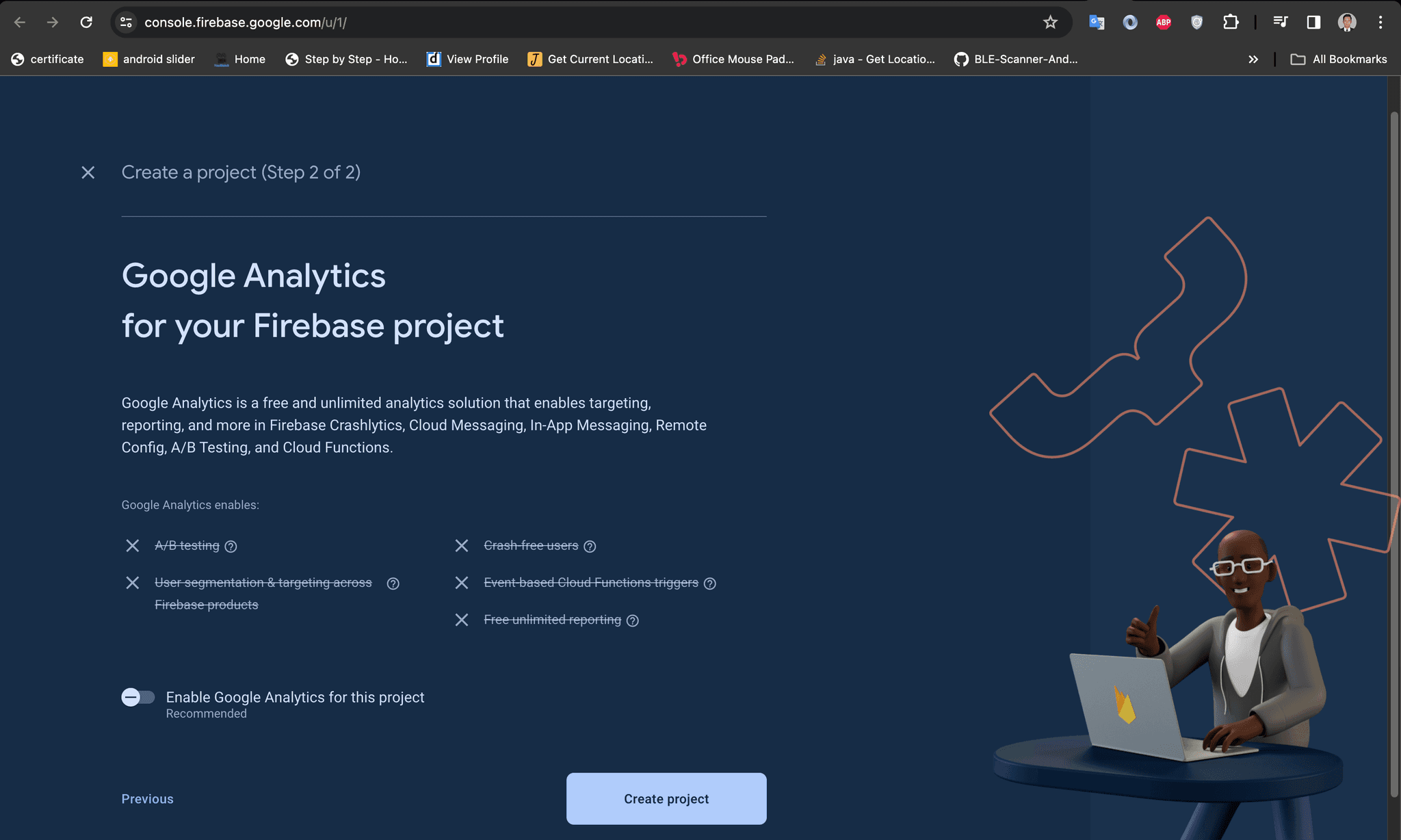
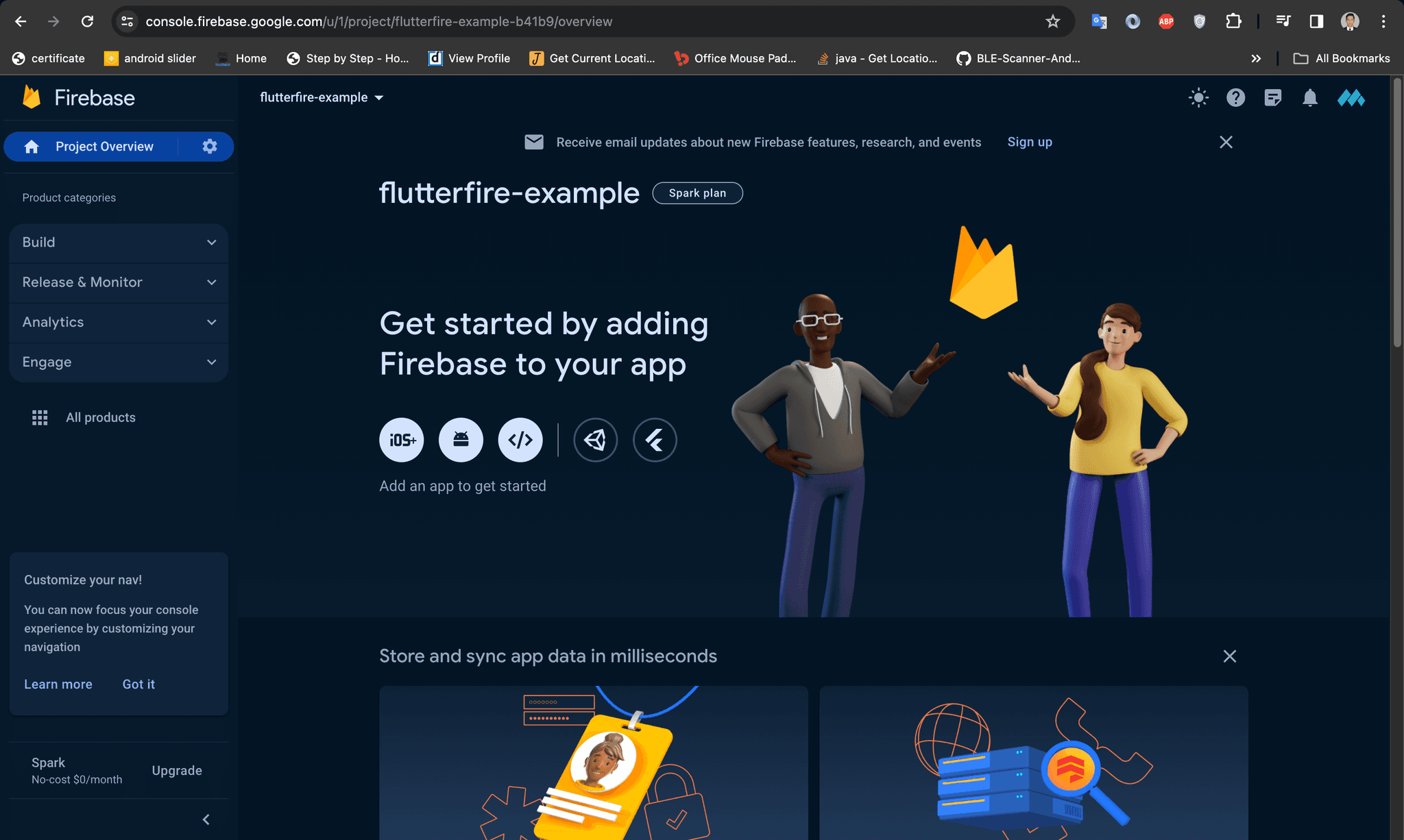
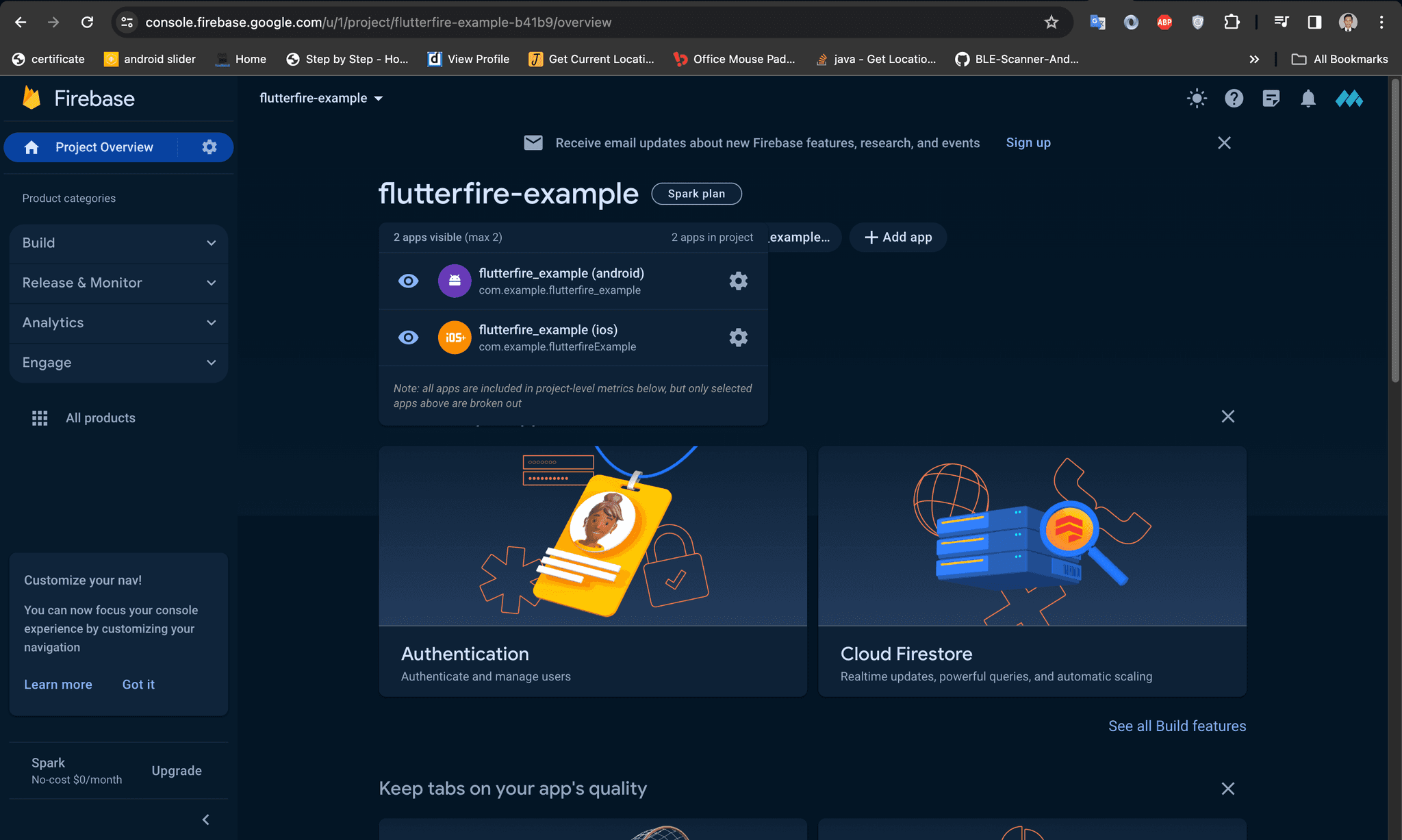
All done in this section. Just leave it and we move to FlutterFire CLI.
3. FlutterFire CLI Installation
Before install FlutterFire, make use you have node / npm in your environment. because we need it to install FlutterFire. check your node like below.
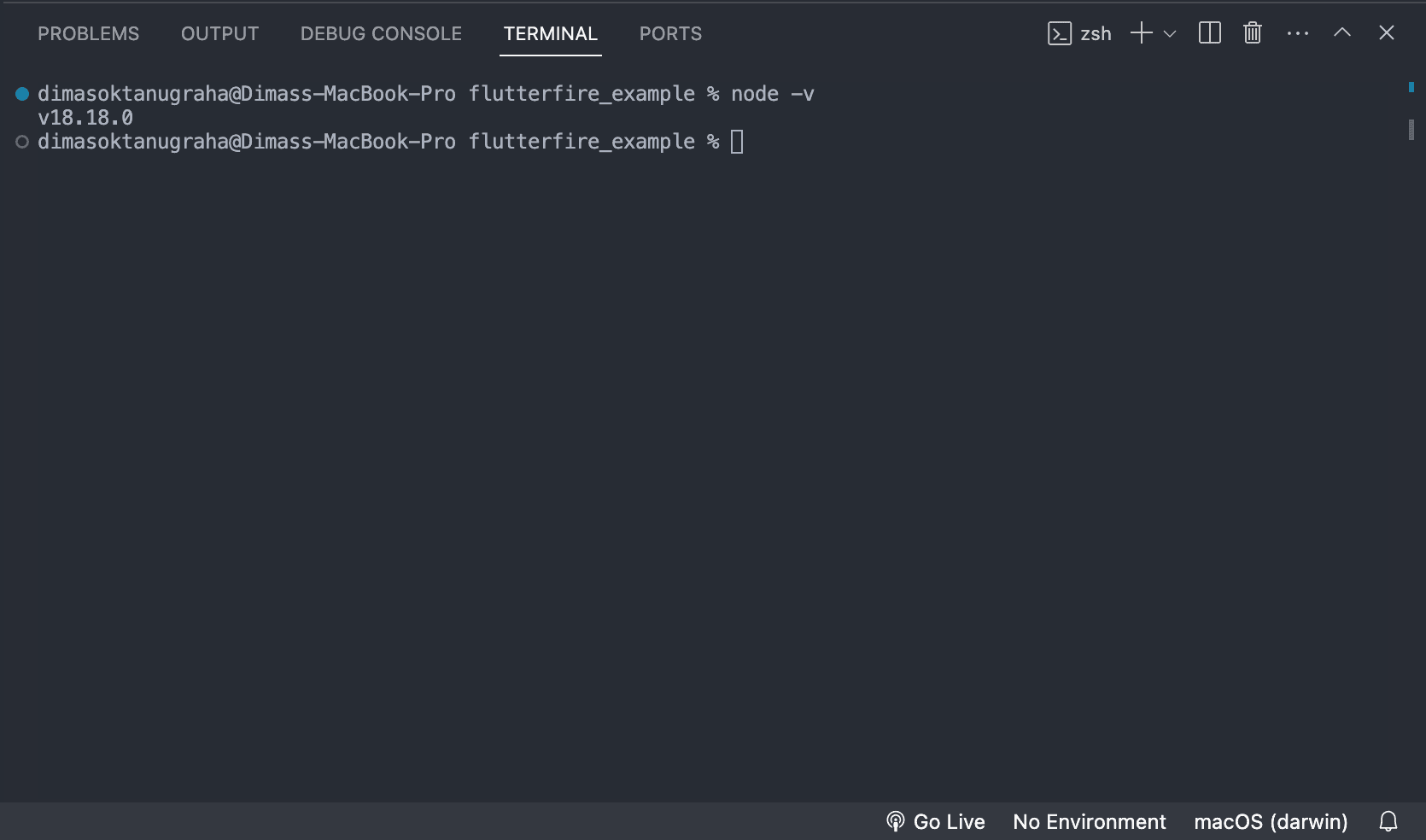
Open your flutter project. in my case, i use vs code and its terminal. Do this step in your terminal:
sudo npm install -g firebase-tools
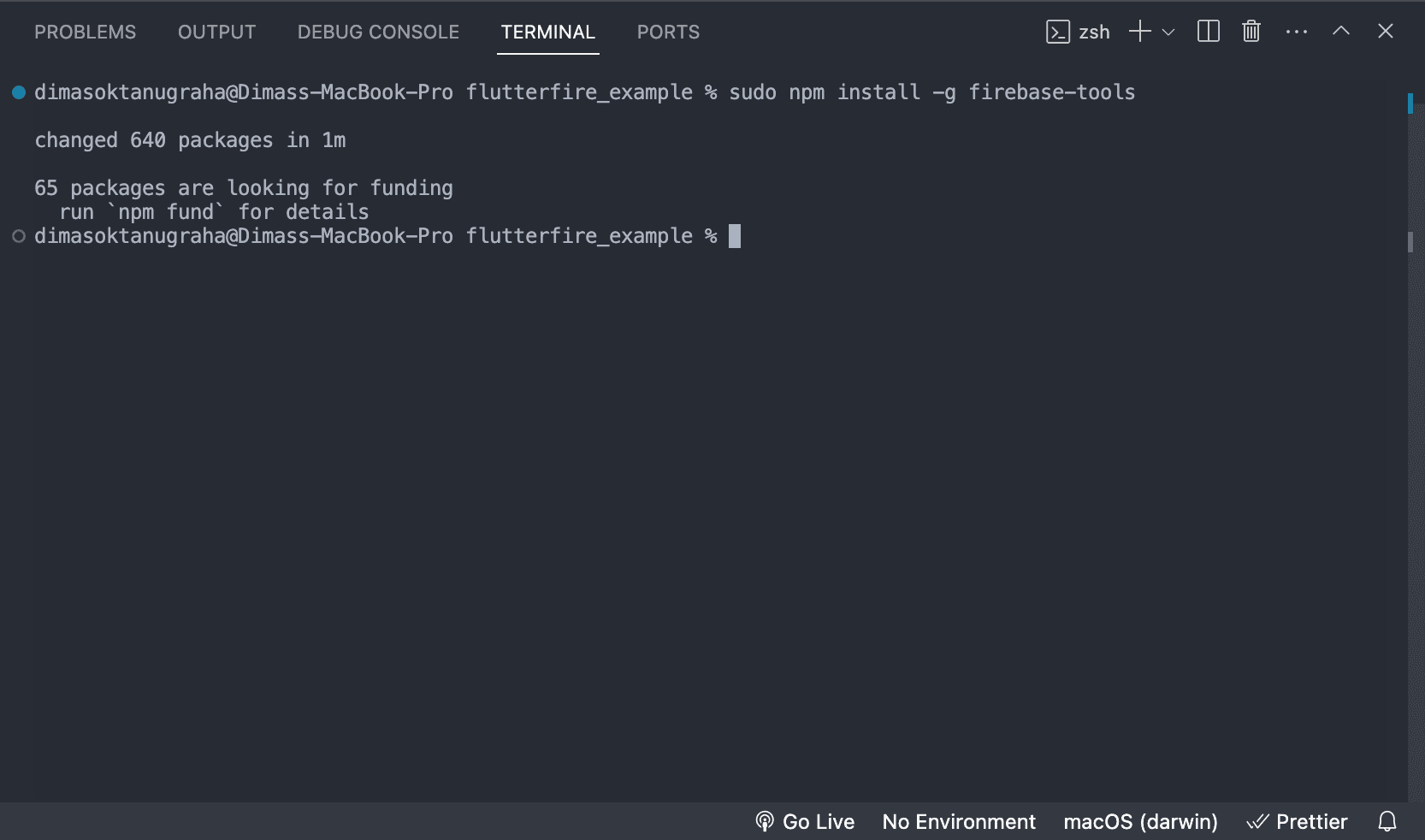
firebase —version
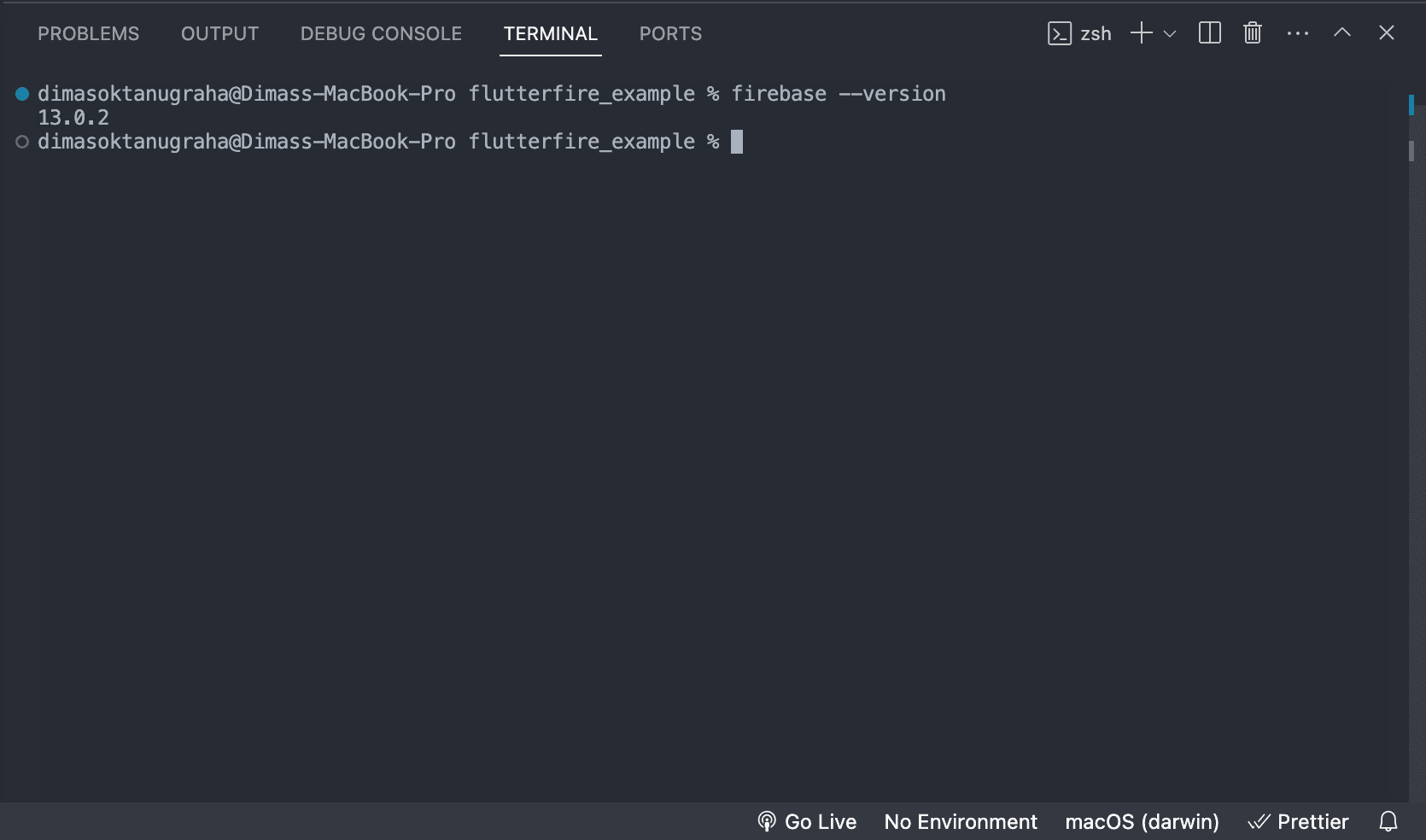
dart pub global activate flutterfire_cli
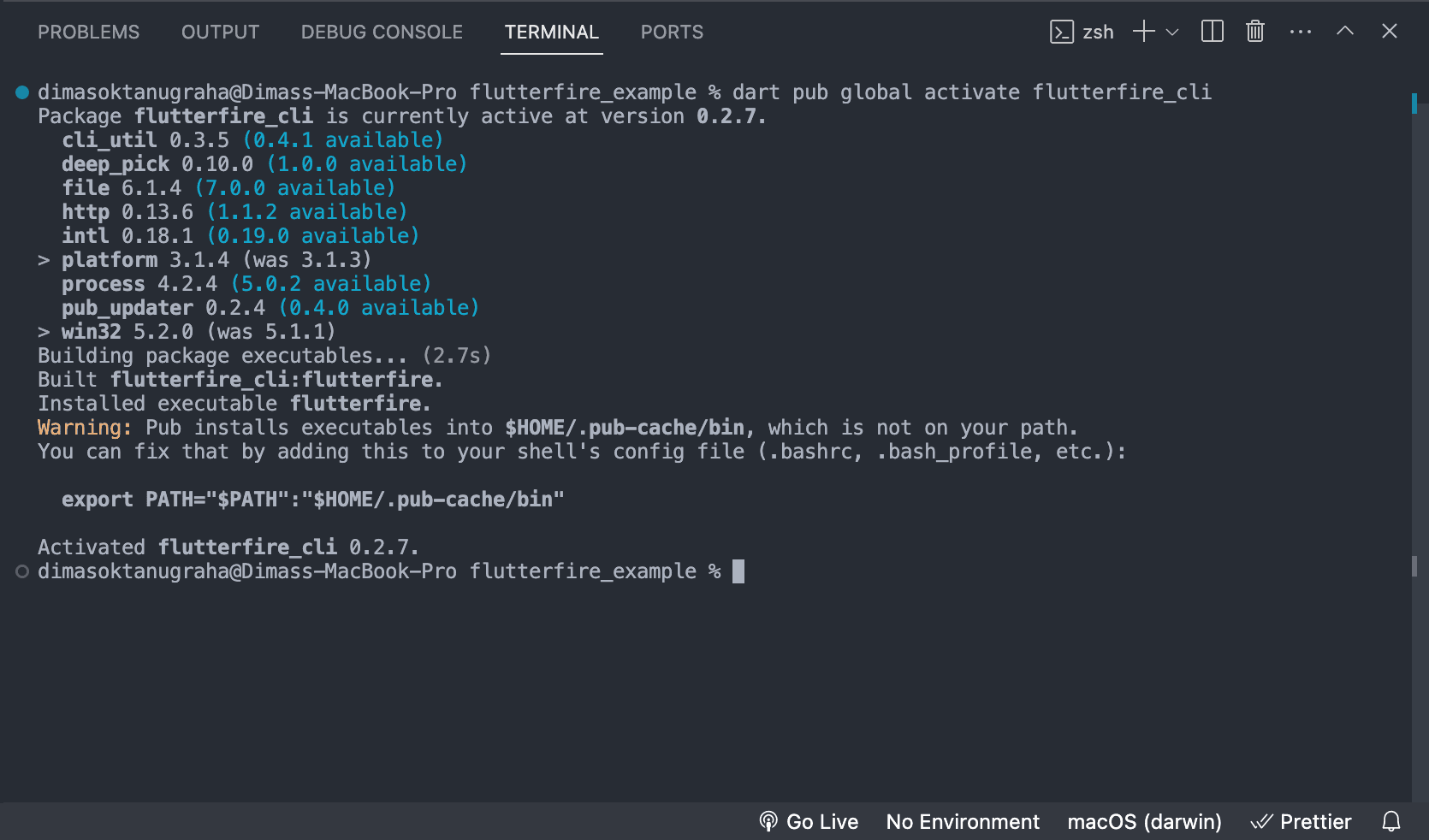
export PATH="$PATH":"$HOME/.pub-cache/bin"
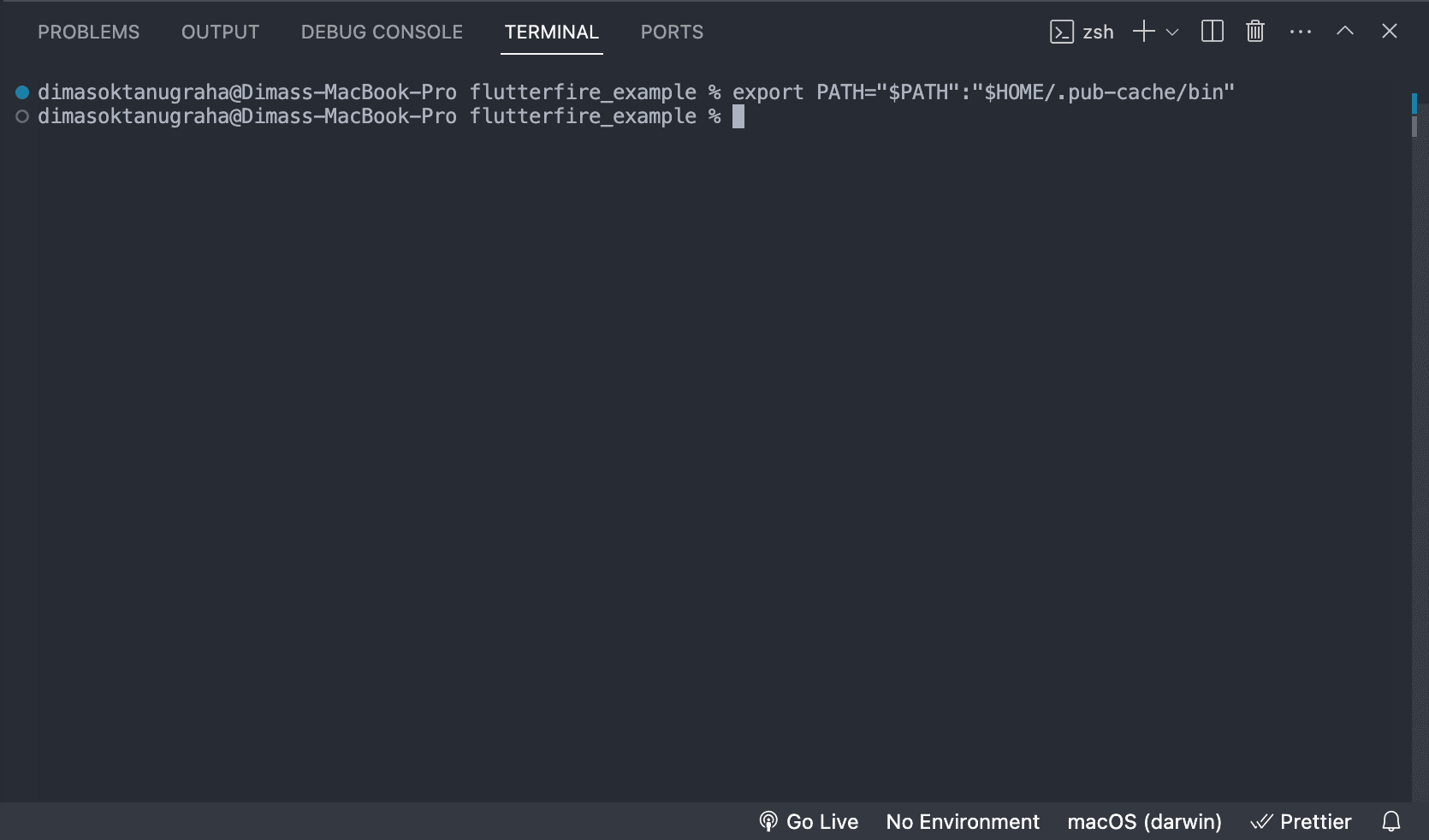
4. FlutterFire Login to Firebase Account
FlutterFire needs to know your firebase account. So we need in login to firebase account through flutterfire CLI.
firebase login
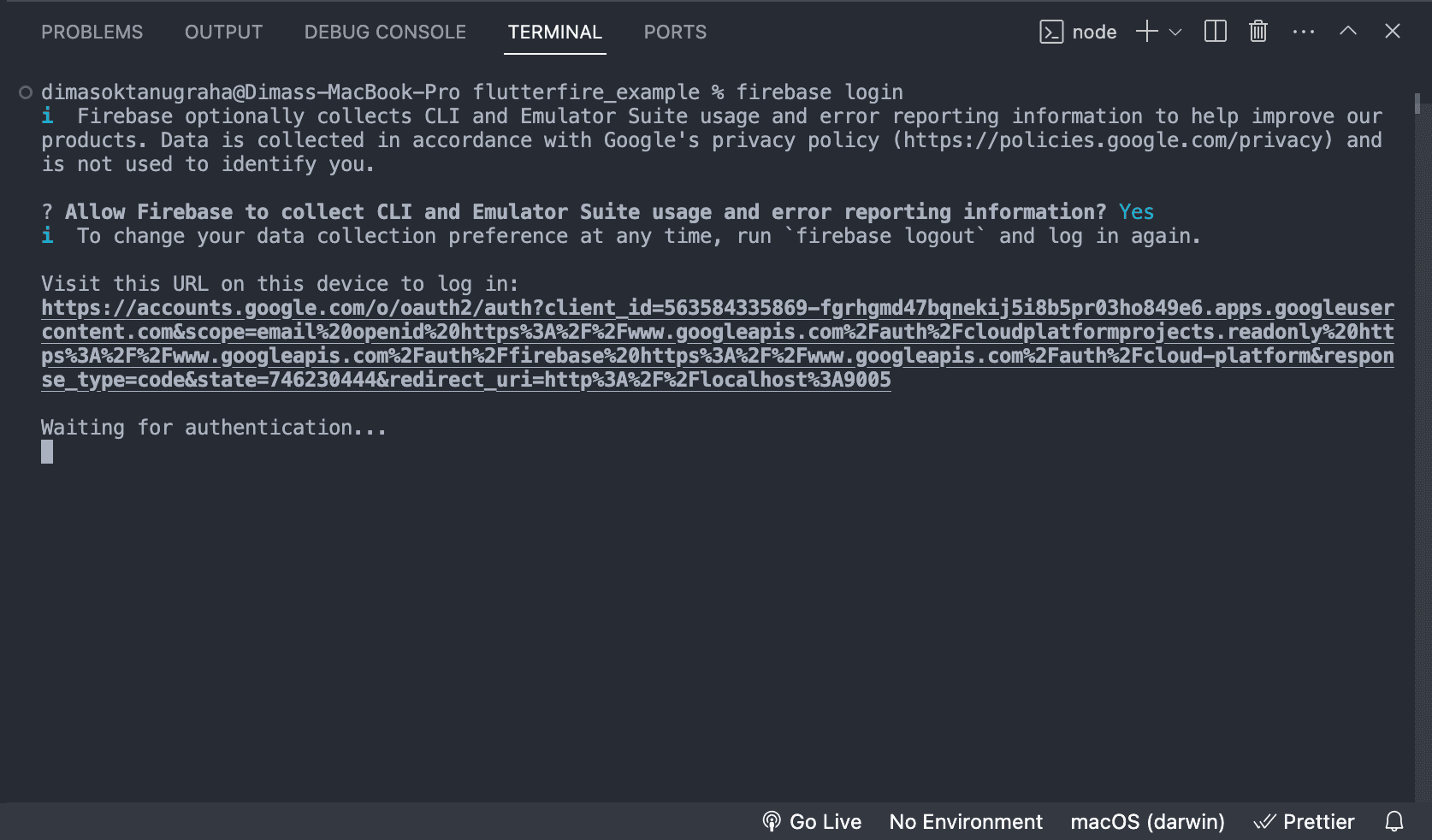
wait for a second and browser will automatically open the link.
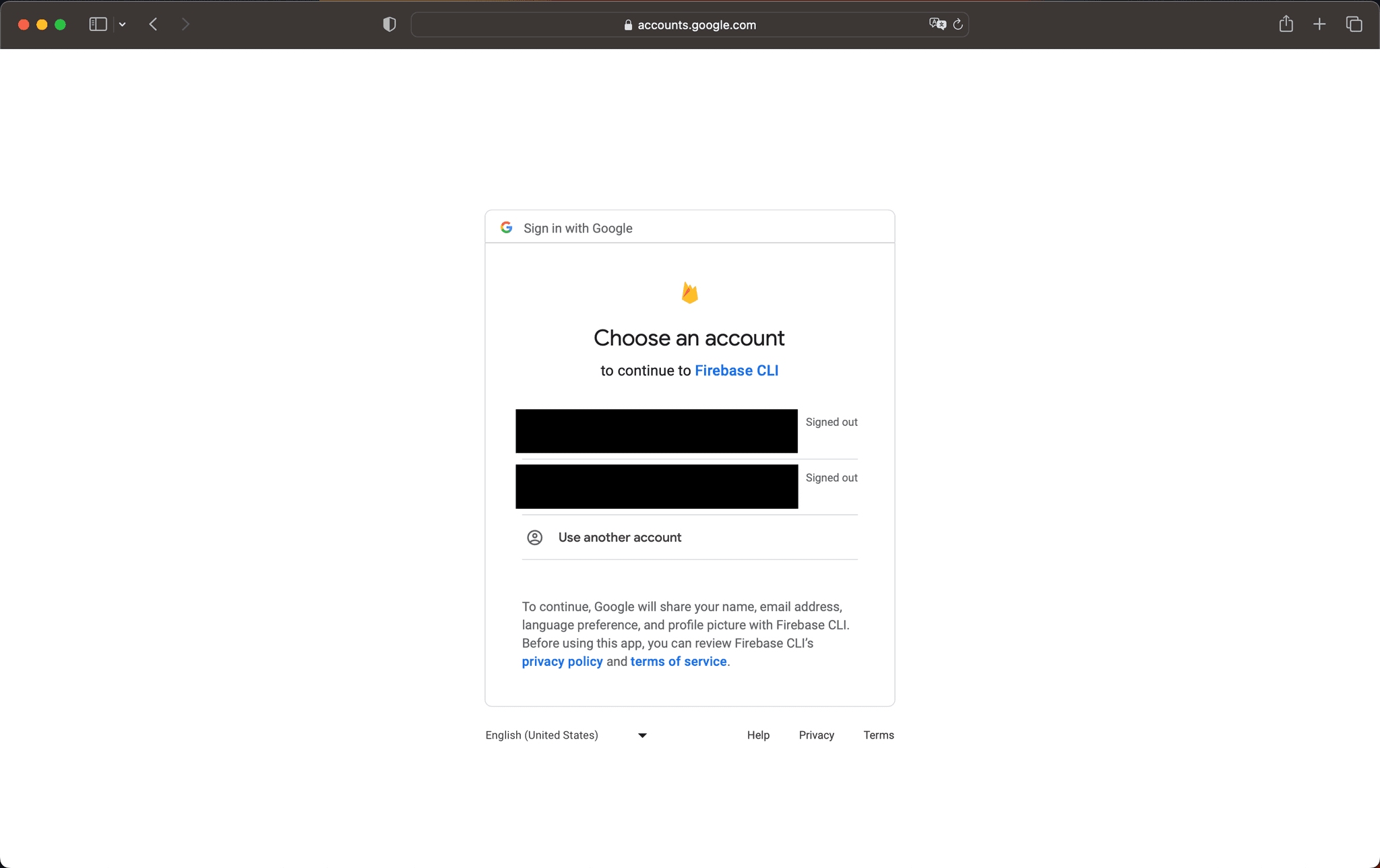
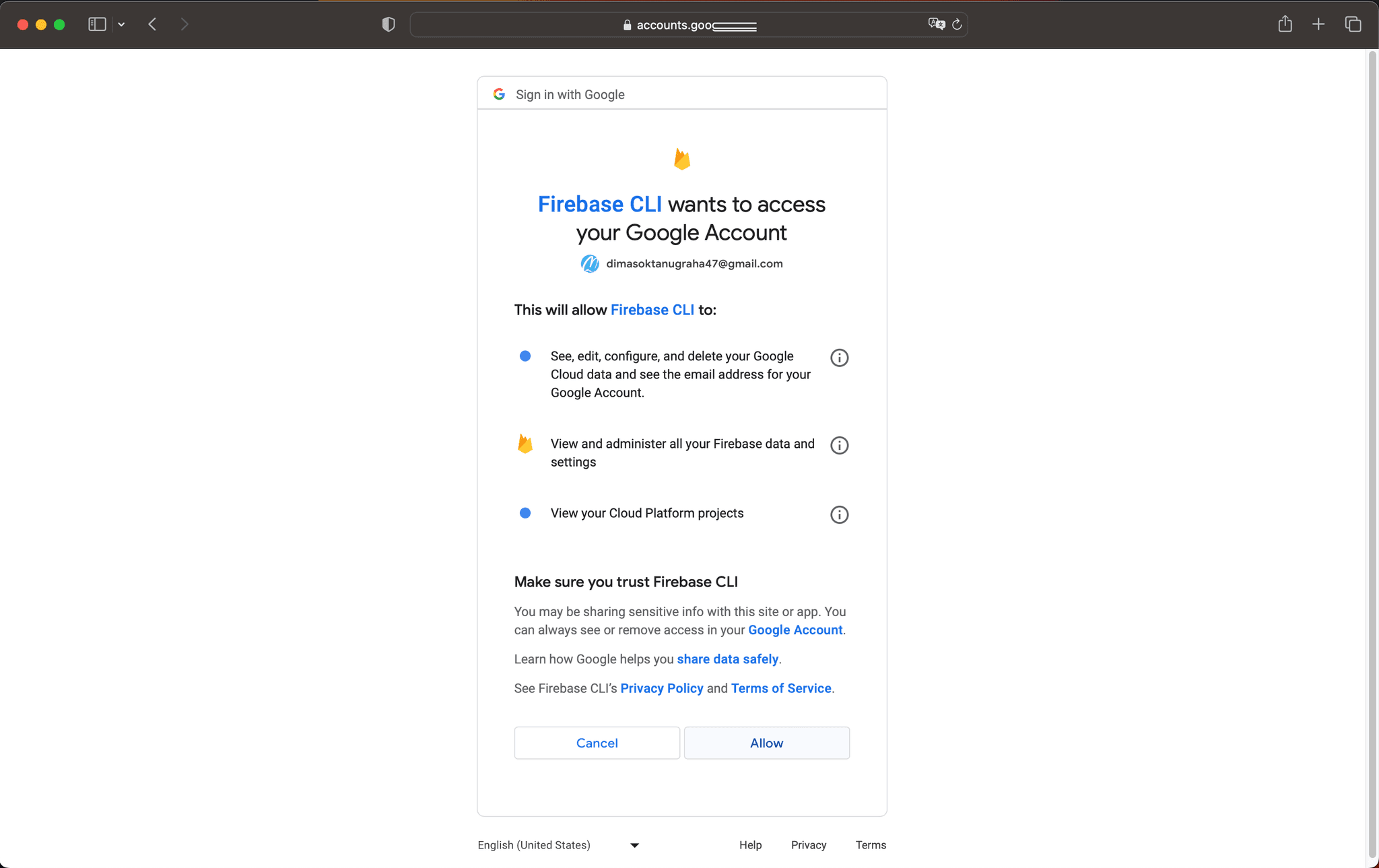
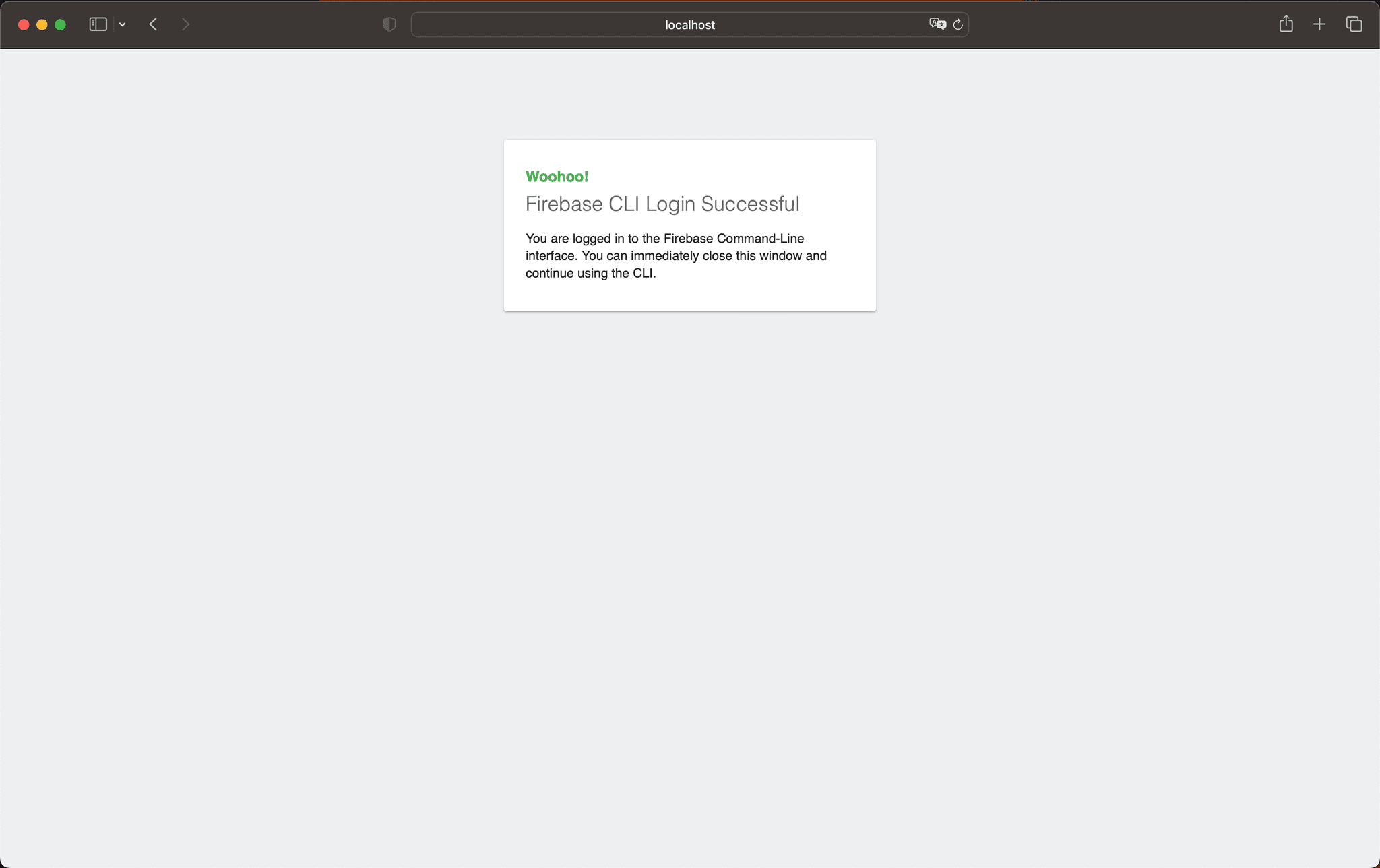
If success like below, flutterfire ready to use.
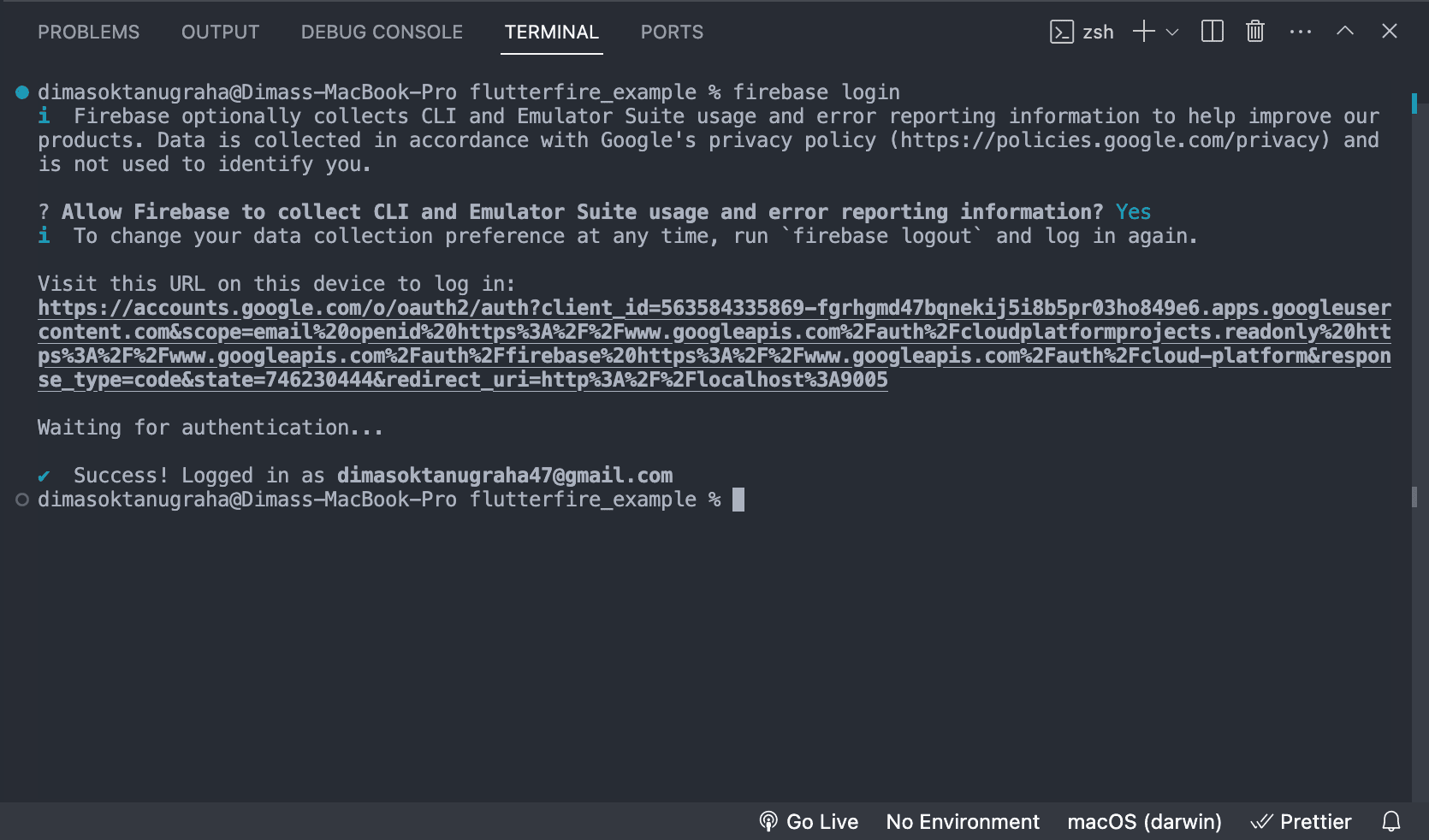
5. Integrate Flutter Project to FlutterFire
Your FlutterFire CLI has installed and you can use it in your flutter project
flutterfire configure
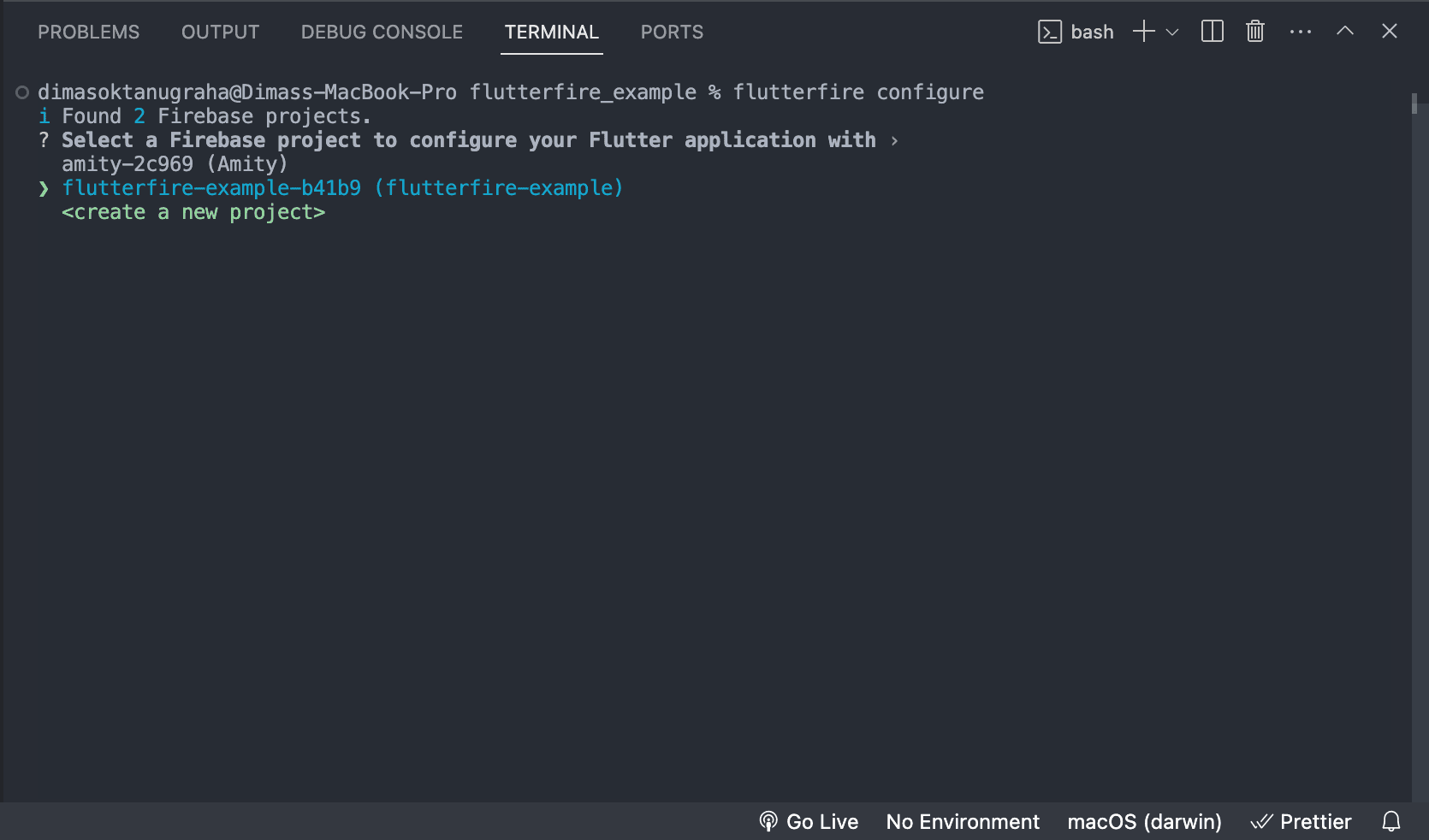
Select your firebase project which you created in step 1.
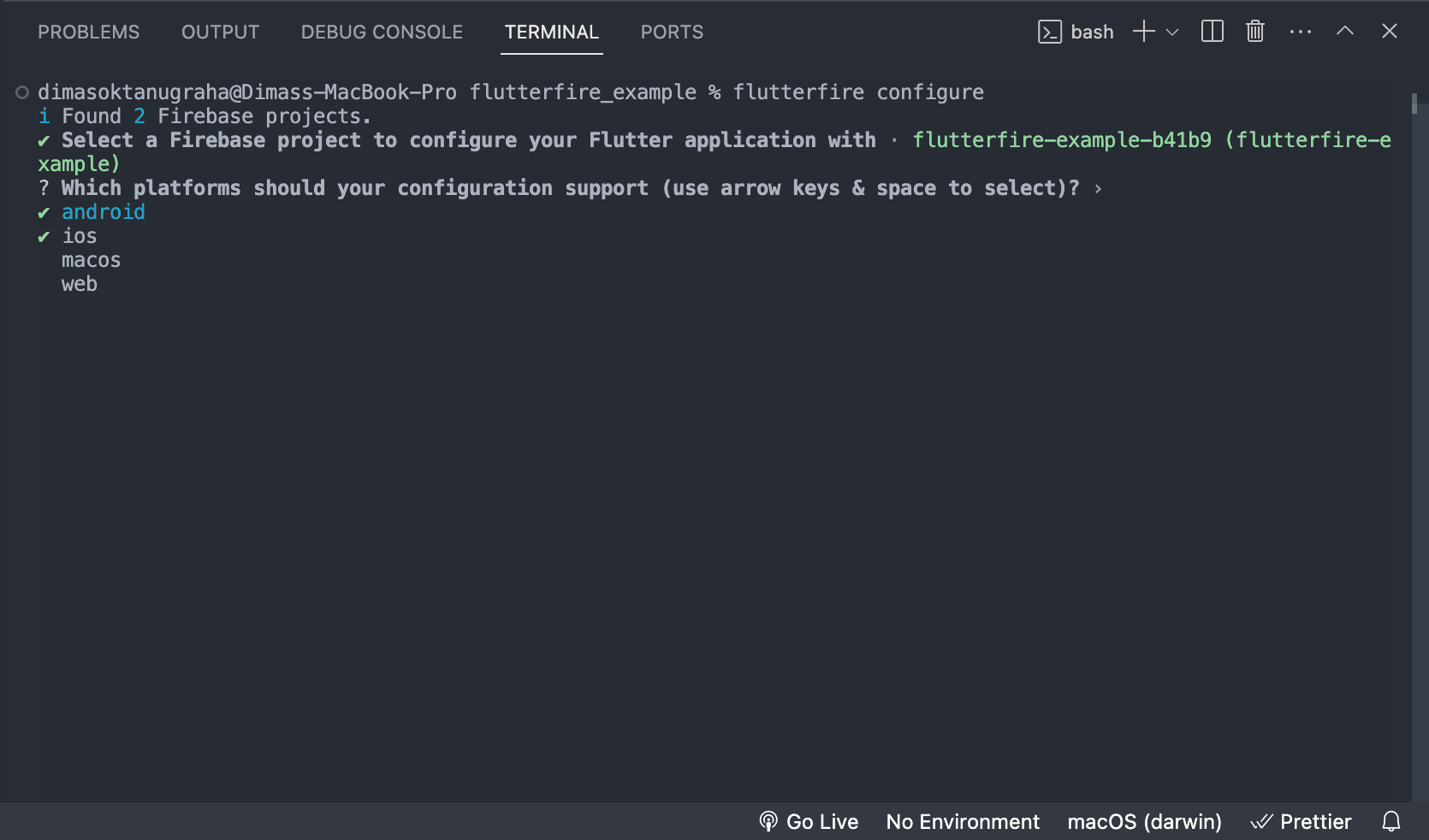
Choose platform that you want to configure and create in your firebase project. in sthis step i choose android and ios ( choose what you want ). Use space to select and unselect.
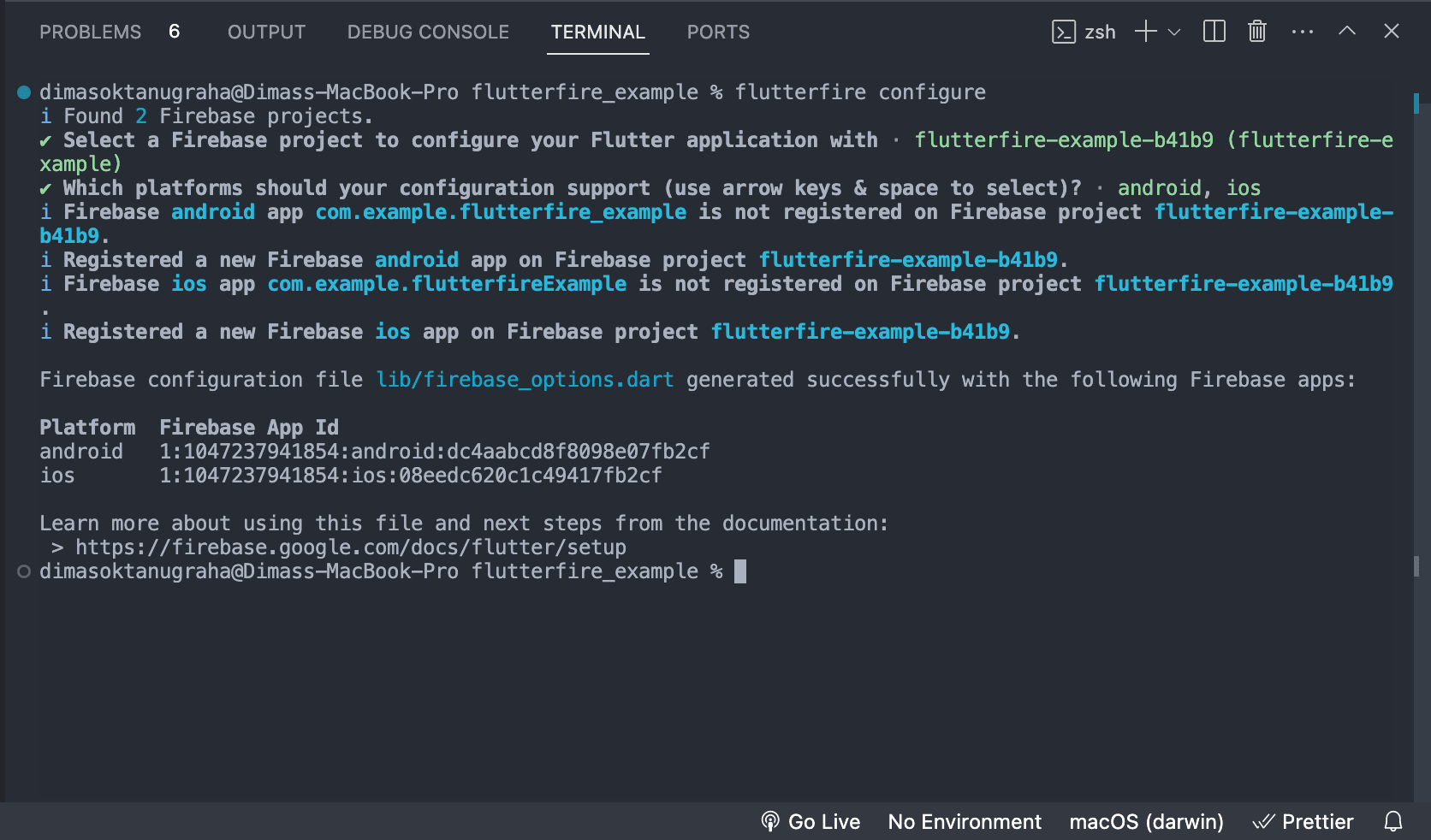
Your Flutter Project already integrated. Before you run the project you need to install firebase-core
flutter pub add firebase_core
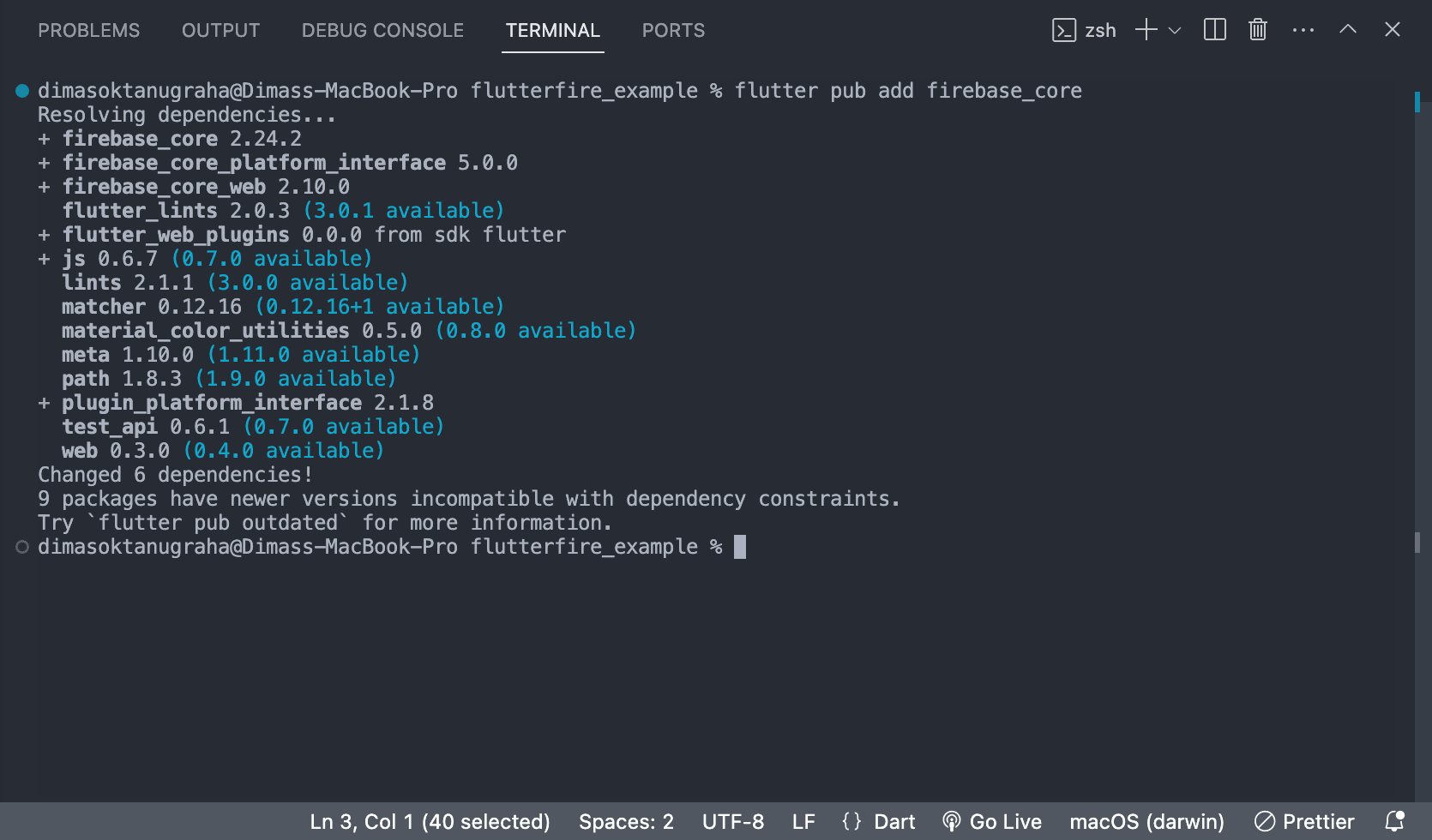
6. Flutter Configuration
After above step, in your flutter project will created a new file 'firebase-option.dart'. this is a proof that you do great job in this integration.
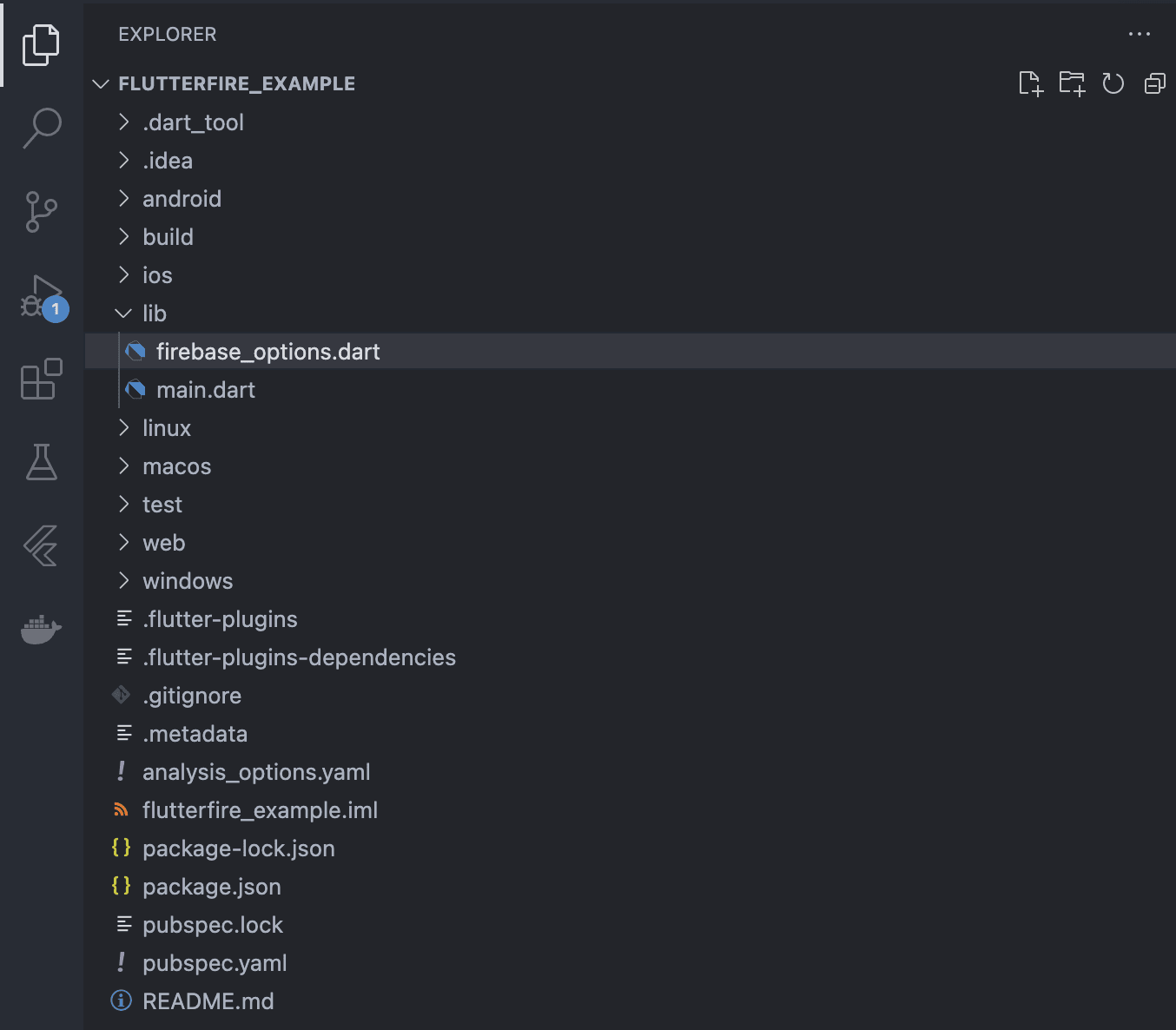
Flutter Core has been installed too.
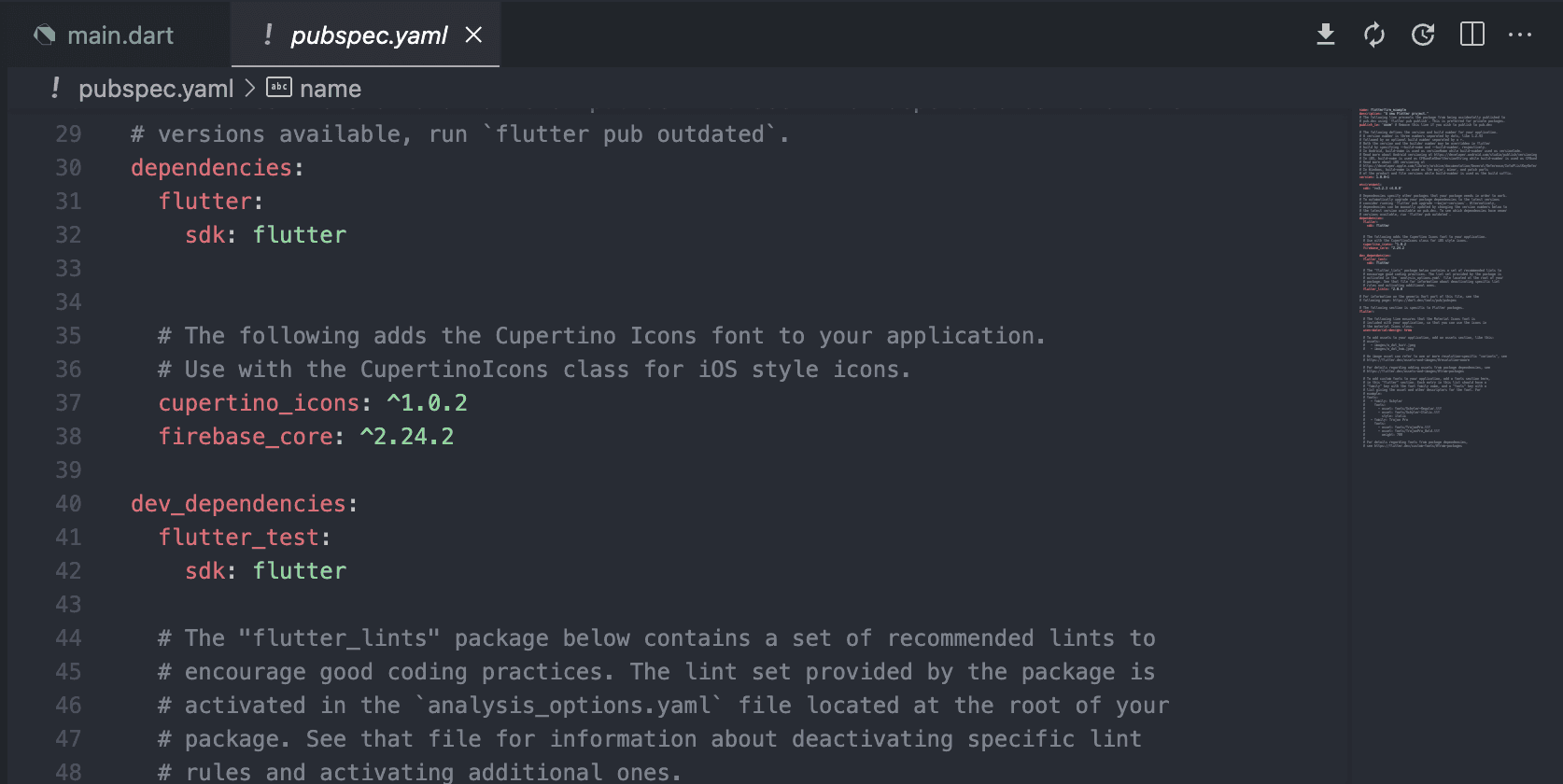
Before you run your project, you need some small task to add some line of code in main.dart.
import 'package:firebase_core/firebase_core.dart';
import 'package:flutter/material.dart';
import 'firebase_options.dart';
Future<void> main() async {
WidgetsFlutterBinding.ensureInitialized();
await Firebase.initializeApp(options: DefaultFirebaseOptions.currentPlatform);
runApp(const MyApp());
}
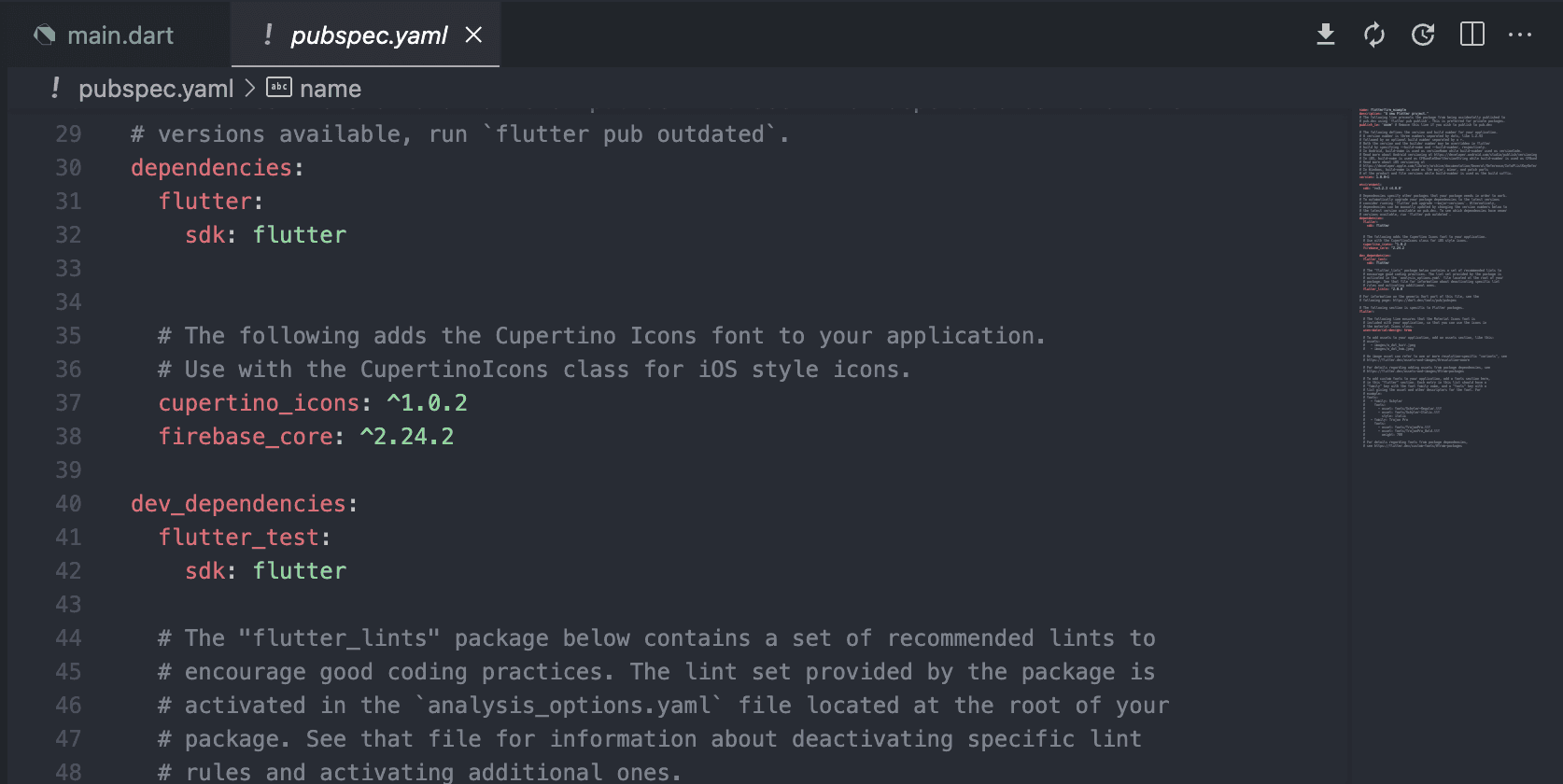
GREAT JOB!
Done for this integration. you can add another firebase feature in your flutter project. for example like below command
flutter pub add firebase_analytics
flutter pub add firebase_auth
flutter pub add cloud_firestore
flutter pub add firebase_database
flutter pub add firebase_storage
flutter pub add firebase_messaging
flutter pub add firebase_crashlytics
flutter pub add firebase_in_app_messaging
flutter pub add firebase_performance
flutter pub add firebase_ml_model_downloader
flutter pub add firebase_remote_config
flutter pub add firebase_app_check
If you want to explore more about FlutterFire CLI, just open their website