Android Compose : Texfield
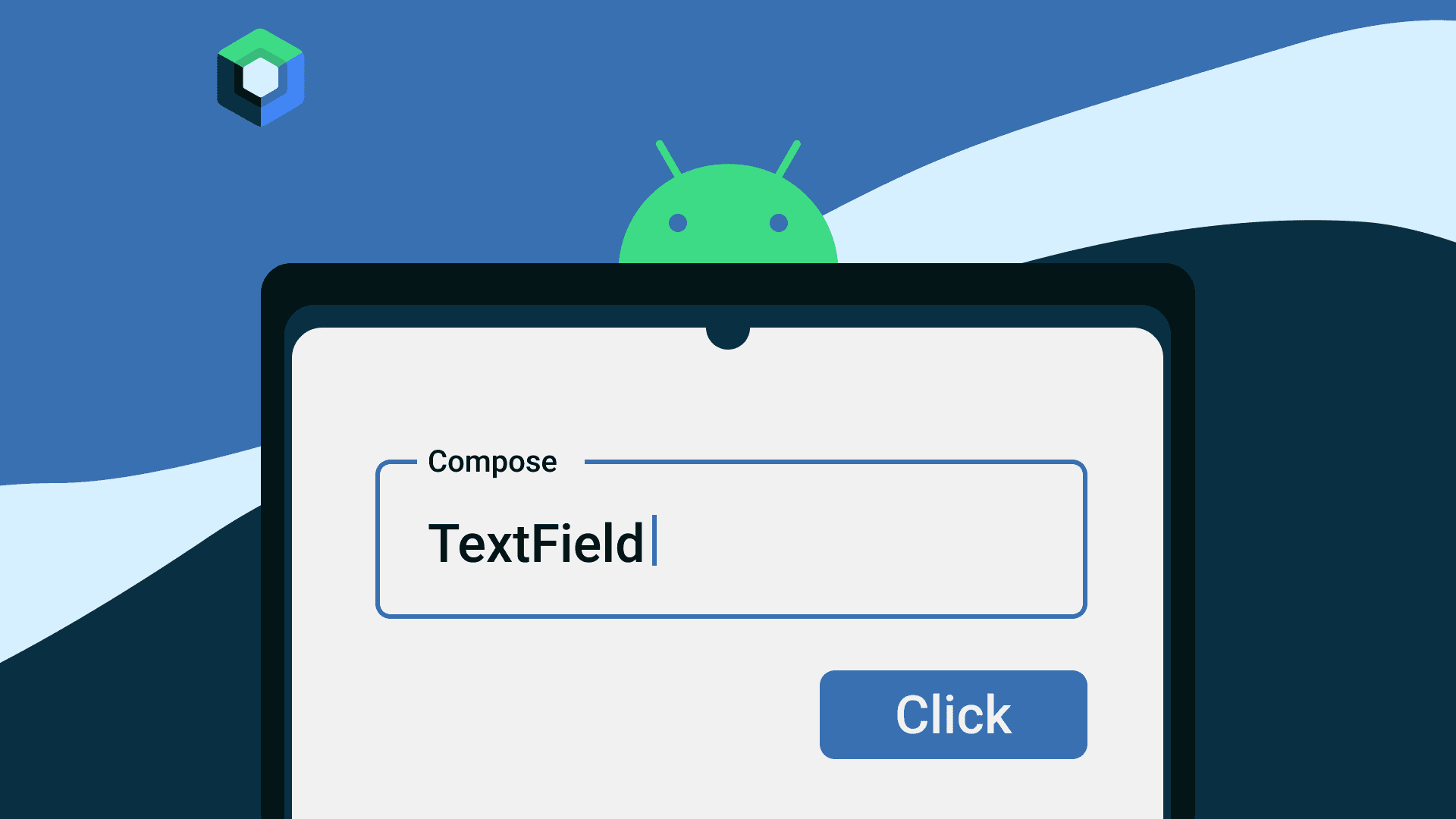
Textfield is a UI component that handle input from user like character, text, number, etc. For Android developer who had experienced with UI system like View or xml known it as EditText
. The newest UI library from jetpack is Compose
which has same behavior like create UI in flutter that as khown as Widget and use state to set and get value from TextField
. The way to implement and use this TextField
is totally different from EditText
like behavior, lifecycle, styling and code.
Here is the simple code that you can use for implementing textfield for the first time:
Simple TextField
@OptIn(ExperimentalMaterial3Api::class)
@Composable
fun TextFieldScreen() {
var text by remember { mutableStateOf("") }
TextField(
value = text,
onValueChange = { newText ->
text = newText
},
label = { Text(text = "Simple") },
placeholder ={ Text(text = "Simple") },
)
}
Output
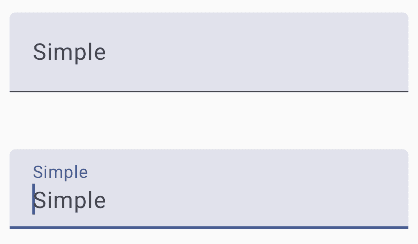
As i mention before, setter and getter in TextField
is different from XML that use ID of EditText View and handle itu from code or XML. For TextField
we must use state like other frontend technology. The code below is state tah handle set and get for TextField
var text by remember { mutableStateOf("") }
TextField(
value = text,
onValueChange = { newText ->
text = newText
},
...
)
value
is where to set data to textfield. So, Textfield will show text based on valueonValueChange
will return string what user input or type and we must save it to statemutableStateOf
is an observable state that save mutable data and change widget when data changed
OutlineTextField
There is another type or design of TextField
with border or outline around it. Here is the code :
@OptIn(ExperimentalMaterial3Api::class)
@Composable
fun TextFieldScreen() {
var text by remember { mutableStateOf("") }
OutlinedTextField(
value = text,
onValueChange = { newText ->
text = newText
},
label = { Text(text = "Outline") },
placeholder ={ Text(text = "Outline") },
)
}
Output
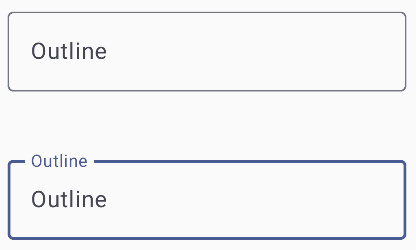
TextField with View Model
Almost every development in Android using View Model to put code about data, observable, variable, fetch API or database, function except for UI and etc. In Compose you can set mutableState inside ViewModel and call it in compose UI. It will make your screen / UI code file clean from another function and variable. Here is the code :
class TextFieldViewModel: ViewModel() {
var text by mutableStateOf("")
private set
fun setValue(value: String){
text = value
}
}
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
val viewModel: TextFieldViewModel by viewModels()
setContent {
ModtionProjectTheme {
TextFieldScreen(viewModel)
}
}
}
}
@OptIn(ExperimentalMaterial3Api::class)
@Composable
fun TextFieldScreen(
viewModel: TextFieldViewModel
) {
OutlinedTextField(
value = viewModel.text,
onValueChange = viewModel::setValue,
label = { Text(text = "Outline") },
placeholder ={ Text(text = "Outline") },
)
}
onValueChange = viewModel::setValue
is same as
onValueChange = { text -> viewmode.setValue(text)}
TextField with Icon
OutlinedTextField(
...
leadingIcon = {
Icon(
imageVector = Icons.Rounded.Lock,
contentDescription = "Leading Icon",
tint = Color.Blue,
modifier = Modifier
.size(24.dp)
)
},
trailingIcon = {
Icon(
imageVector = Icons.Rounded.Close,
contentDescription = "Trailing Icon",
tint = Color.Blue,
modifier = Modifier
.size(24.dp)
.clickable {}
)
},
)
Output
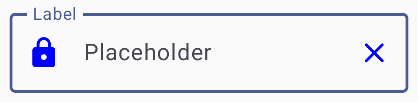