Android : Build Types and Product Flavors
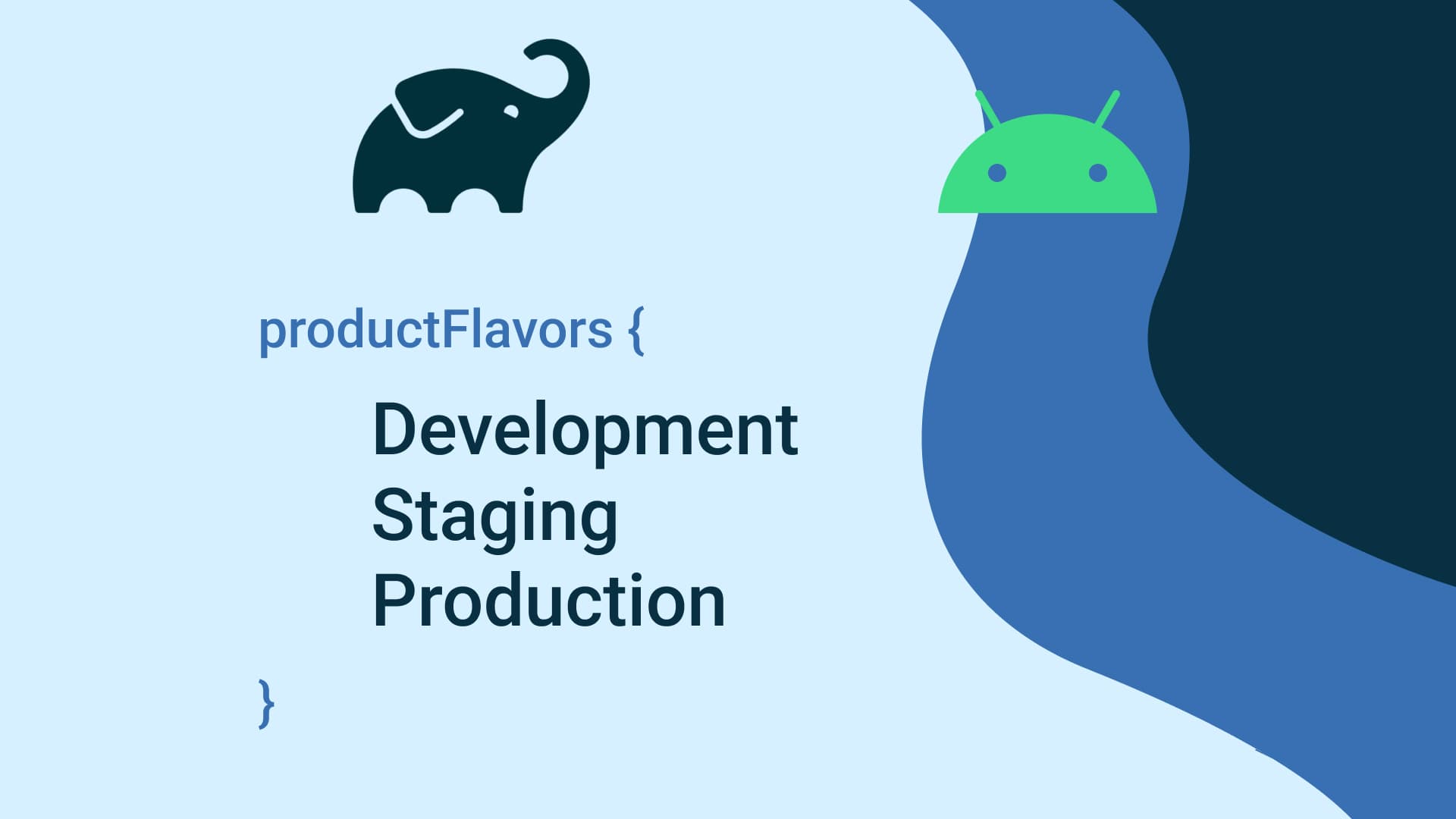
Build Types
For default setting in build types just has 2 types, debug and release. debug
is for development environment and can create the build without any setting. release
build is meant to uploaded to Play Store, need to Signing APK and obfuscate to prevent reverse-engineering. Size of release build is smaller than debug.
Default Build Type
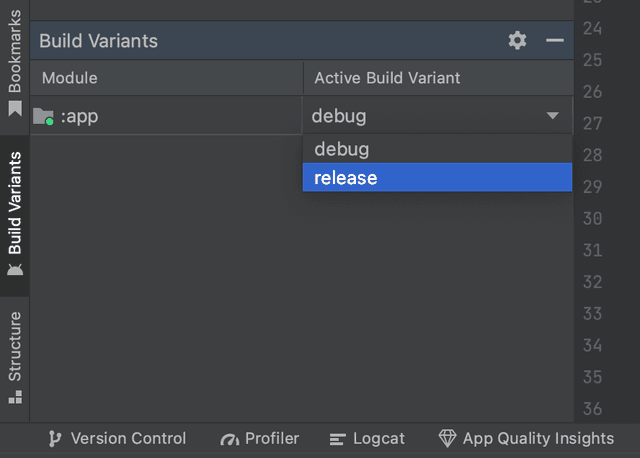
You can create new build types in app/build.gradle.kts
. Default code in buildTypes just for release
buildTypes {
release {
isMinifyEnabled = false
proguardFiles(
getDefaultProguardFile("proguard-android-optimize.txt"),
"proguard-rules.pro"
)
}
}
Let's add debug
and add some option. we will add suffix like code below and change package app name.
buildTypes {
release {
isMinifyEnabled = false
isShrinkResources = true
proguardFiles(
getDefaultProguardFile("proguard-android-optimize.txt"),
"proguard-rules.pro"
)
}
debug {
applicationIdSuffix = ".debug"
versionNameSuffix = "-debug"
}
}
Product Flavors
Android can create many environment and build types. It has purpose to separate some apps. In IT software development, there are same apps but with different function:
Development
apps for development function and just for developerStaging
apps for Testing Environment that will used by QA and reported it to developer if it has some bugsProduction
apps is final apps for real user that will released to Play Store
android {
flavorDimensions += "env"
productFlavors {
create("development") {
dimension = "env"
}
create("production") {
dimension = "env"
}
}
}
The result of this code is create 4 build types/variant. Each Flavors will have each build types. Here is the result:
Result Product Flavors
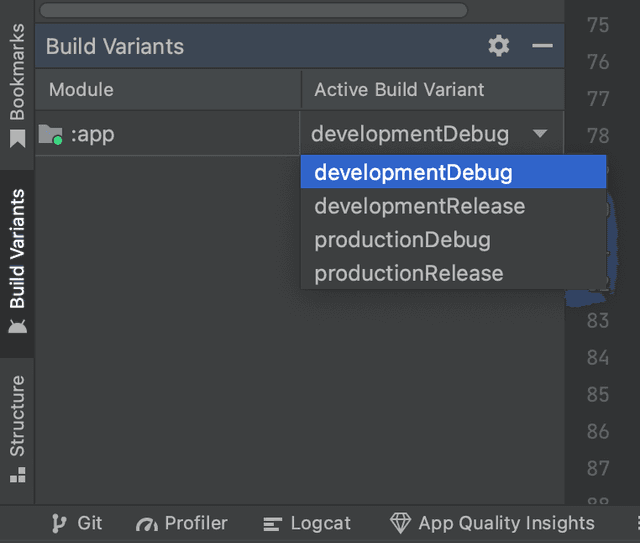
We can use flavors to create different data in each environment. for example base URL API, in many case API URL can be different to prevent real data from user and data for development not mixed with each other also used of different server. we can set the API URL like code below and we must add buildConfig = true
inside buildFeatures
to inform the gradle to generate buildConfigField that we create.
android {
...
buildFeatures {
...
buildConfig = true
}
...
flavorDimensions += "env"
productFlavors {
create("development") {
dimension = "env"
buildConfigField("String", "API_URL", "http://api.development.com")
}
create("production") {
dimension = "env"
buildConfigField("String", "API_URL", "http://api.development.com")
}
}
}
Call BuildConfig.API_URL
in every place that you need especially in Retrofit Builder. The API URL will adjust to the build type you choose. Flavors are not only used to create environments, we can create various types according to tech requirement. Here is the example if you want to make free and pro apps to handle your admob ads.
android{
...
val additionalFlavor = listOf("env", "type")
flavorDimensions += additionalFlavor
productFlavors {
create("development") {
dimension = "env"
buildConfigField("String", "API_URL", "http://api.development.com")
}
create("production") {
dimension = "env"
buildConfigField("String", "API_URL", "http://api.development.com")
}
create("free") {
dimension = "type"
applicationIdSuffix = ".free"
versionNameSuffix = "_free"
buildConfigField("Boolean", "SHOW_ADS", "true")
}
create("pro") {
dimension = "type"
buildConfigField("Boolean", "SHOW_ADS", "false")
}
}
}
Result Type Flavors
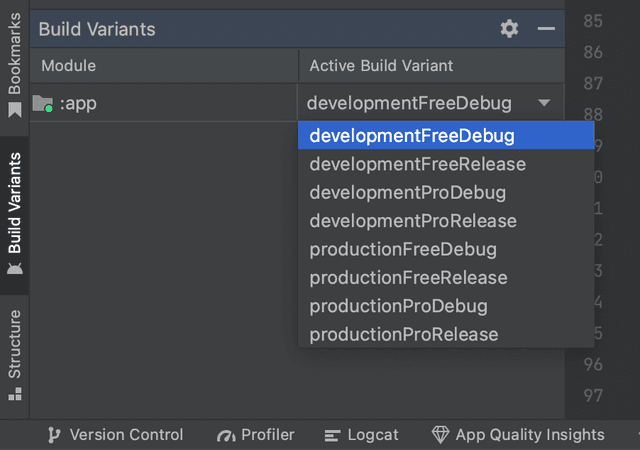